Day 9, Year 2015: All in a Single Night
First read the problem description.
import networkx as nx
def draw(g):
= nx.spring_layout(g, k=g.number_of_nodes()*4)
pos =pos,
nx.draw(g, pos=True,
with_labels=4500,
node_size=4)
width= nx.get_edge_attributes(g, 'weight')
labels
nx.draw_networkx_edge_labels(g, pos,=labels)
edge_labels
def possibile_paths(g, r, cn, paths, total_weight=0):
'visited'] = True
g.nodes[cn][
= True
last_node for v, ed in g.adj[cn].items():
if not g.nodes[v].get('visited'):
= False
last_node
possibile_paths(g, r, v,
paths,=total_weight+ed['weight'])
total_weight
if last_node == True:
paths.append((r, cn, total_weight))
'visited'] = False
g.nodes[cn][
def all_paths(g):
= []
paths for n in g.nodes:
possibile_paths(g, n, n, paths)
return paths
= nx.Graph()
g 'London', 'Dublin', 464),
g.add_weighted_edges_from([('London', 'Belfast', 518),
('Dublin', 'Belfast', 141)])
(
draw(g) all_paths(g)
[('London', 'Belfast', 605),
('London', 'Dublin', 659),
('Dublin', 'Belfast', 982),
('Dublin', 'London', 659),
('Belfast', 'Dublin', 982),
('Belfast', 'London', 605)]
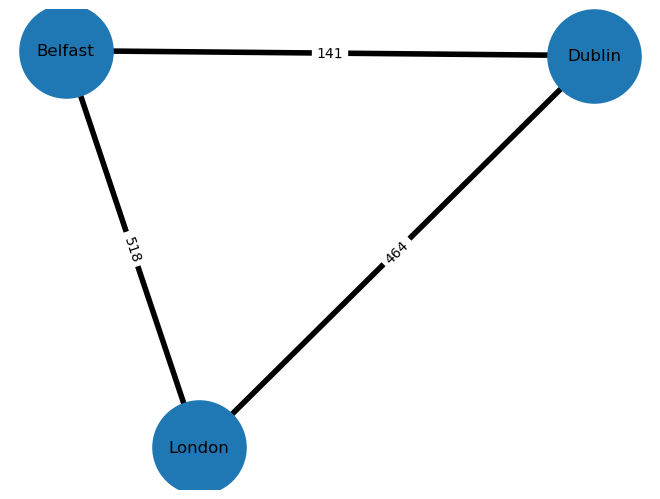
= nx.Graph()
g # I'm too lazy to parse a file
'AlphaCentauri', 'Snowdin', 66),
g.add_weighted_edges_from([('AlphaCentauri', 'Tambi', 28),
('AlphaCentauri', 'Faerun', 60),
('AlphaCentauri', 'Norrath', 34),
('AlphaCentauri', 'Straylight', 34),
('AlphaCentauri', 'Tristram', 3),
('AlphaCentauri', 'Arbre', 108),
('Snowdin', 'Tambi', 22),
('Snowdin', 'Faerun', 12),
('Snowdin', 'Norrath', 91),
('Snowdin', 'Straylight', 121),
('Snowdin', 'Tristram', 111),
('Snowdin', 'Arbre', 71),
('Tambi', 'Faerun', 39),
('Tambi', 'Norrath', 113),
('Tambi', 'Straylight', 130),
('Tambi', 'Tristram', 35),
('Tambi', 'Arbre', 40),
('Faerun', 'Norrath', 63),
('Faerun', 'Straylight', 21),
('Faerun', 'Tristram', 57),
('Faerun', 'Arbre', 83),
('Norrath', 'Straylight', 9),
('Norrath', 'Tristram', 50),
('Norrath', 'Arbre', 60),
('Straylight', 'Tristram', 27),
('Straylight', 'Arbre', 81),
('Tristram', 'Arbre', 90)])
(
draw(g)
= all_paths(g)
paths
import operator
print(min(paths, key=operator.itemgetter(2)))
print(max(paths, key=operator.itemgetter(2)))
('Tristram', 'Arbre', 141)
('AlphaCentauri', 'Faerun', 736)
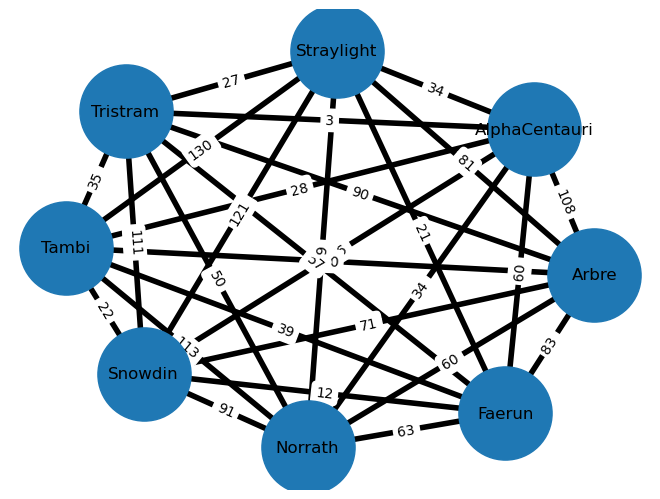
Source code of the solution(s):